We all know about the evil of duplication. Multiple names for exactly the same method can seem like a bad idea, especially if you come from less “dynamic” languages.
A famous alias case that most experienced coders encounter when they dive into ruby: the commonly known reduce
method. In ruby, it's called inject
but you can also use reduce
, they do exactly the same thing.
To make things worse, in Ruby, you can define your custom method names:
irb(main)* class String
irb(main)* alias_method :concatenate, :+
irb(main)> end
irb(main)> 'stone'.concatenate 'rich'
=> "stonerich"
As many as you wish, for any method:
alias :funny_name :critical_method
alias :gufu :critical_method
So, at some point some programmers are like "Oh Lord, why do I have to stuff my brain with all that?" (In his book Refactoring, Martin Fowler admittedly says that he codes in a way, so that he doesn't have to remember things because he's afraid that his brain gets full - mind that 🧠).
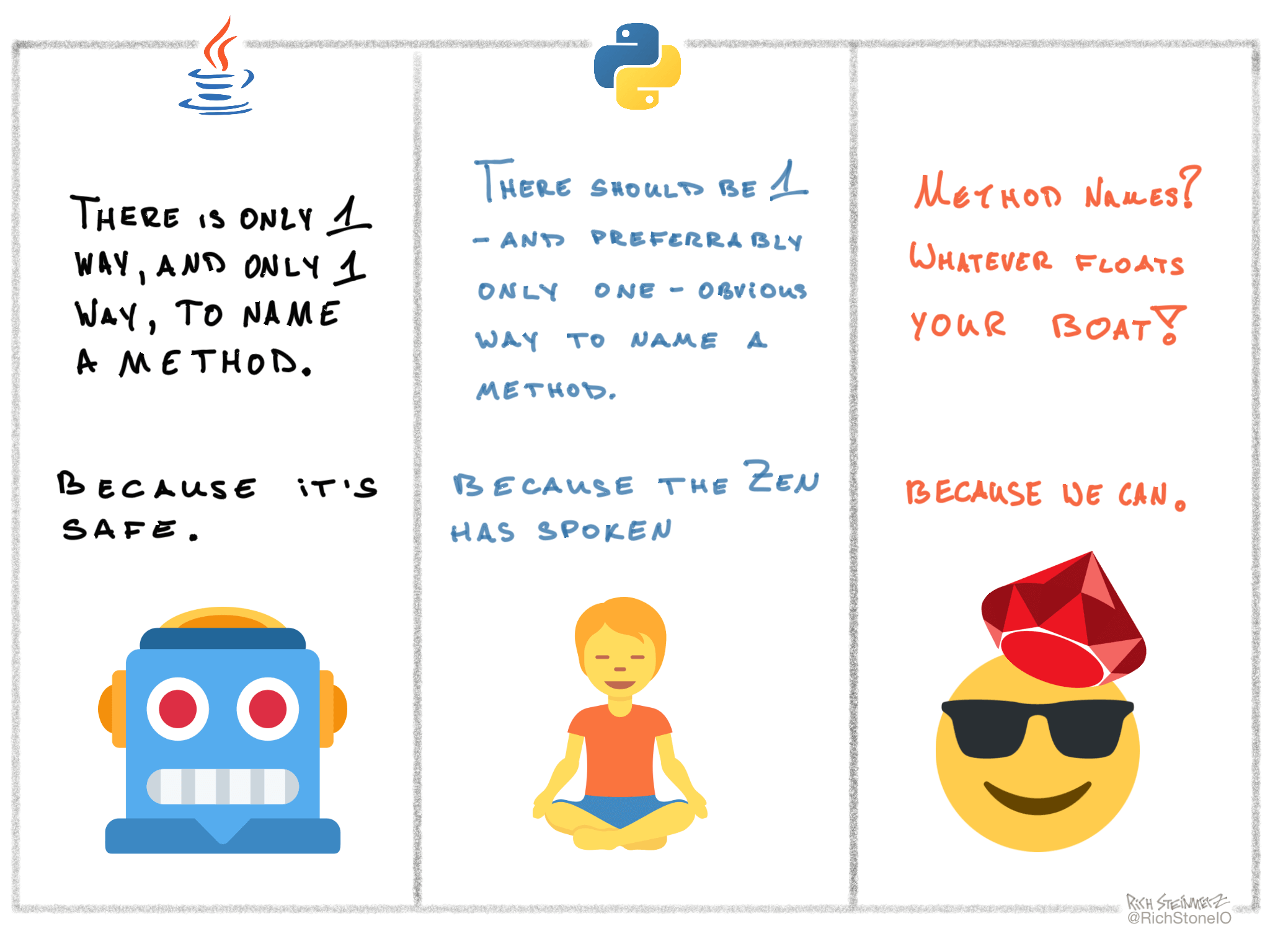
Productivity
In the end, it's all for your own good. Your developer productivity. Here a few common use cases of alias
and alias_method
:
Add spelling variations
Depending on where you grew up, both are fine:
# US people are like...
Office.organize
# ... and then UK people fight back like...
Office.organise
Avoid common misspellings
If you are German, this might have happened to you during your journey of mastering the English language:
class Person
def address
'Burundi Street 5'
end
# better do this with German programmers
# in the team to avoid NoMethodErrors:
alias :adress :address
end
Make coding more intuitive and clear
We communicate with code. Sometimes we have the same functionality but want to express different intents. Or we want to make methods to be more precise than its initial definition allows us to be.
For example, Rspec's describe
and context
do the exact same thing in your code, but they will change how you and others read and write a given spec.
Another example would be Rails' beginning_of_day
method. The method was probably initially defined like this, but then people thought: What's actually a "beginning of a day"? I wake up at 6 am and my day begins, but for my iPhone, the day begins at 00:00.
alias :midnight :beginning_of_day
alias :at_midnight :beginning_of_day
alias :at_beginning_of_day :beginning_of_day
In some contexts, midnight
reads better, in other contexts at_midnight
is a better pick:
date = Birth.date
if Time.now > date.midnight
send_email(when: date.at_midnight)
end
When do aliases become productive and fun?
When the method names flow down your fingers and your code becomes crystal clear, you will see the gains! (Not before googling ruby inject
and inject vs reduce ruby
for the 15th time, though, and not before picking up all the awesome methods in other people's codes).
So far I'm getting around well with all this flexibility and probably my brain has not yet reached its final capacity 🙏
P.S.: The alias for alias
is alias_method
.
Just kidding, there’s a difference. But you shouldn't use either of them anyway. More on this later ;)